2 Basics of Tkinter
Introduction to GUI Programming and Object Oriented Programming Review
Overview
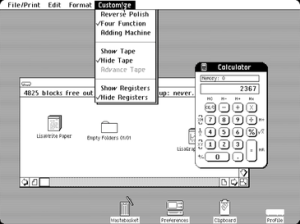
Imagine your computer screen: The windows, icons, buttons, and menus you see and are able to click – that’s your Graphical User Interface, or GUI (pronounced “gooey”).
GUI allows users to interact with their computers using visual elements called widgets – individual elements that make up a GUI such as buttons, icons, menus, search icons etc., thus making the use of computers easy, interactive, and intuitive for everyone.
TEKS Computer Science 1 (3): To foster students’ creativity and innovation by presenting opportunities to design, implement, and present meaningful programs through a variety of media
Content Objective
- Students will create a simple graphical user interface using basic Tkinter widgets, including windows, frames, labels, buttons, entry fields, and textbox areas.
- Students will learn how Tkinter variables work and methods for input validation.
- Keywords (Phrases): Graphical User Interface, Widgets
Language Objective (read, write, speak):
- Students will be able to read and interpret Python code for GUI programming.
- Students will be able to write descriptive code comments explaining the purpose any logic used in their project
- Students will verbally explain the purpose of GUI components and their implementation in Python to their peers.
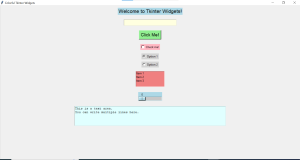
Some Points of Consideration In Building a user Interface
- Layout: The goal here is to arrange elements on the screen to create a logical flow and hierarchy. A well-designed layout helps users quickly find the information they need and understand the relationships between different components. Use Container widgets here to help improve your layout
- Colors: Colors can set the tone for an interface, evoking emotions, and helping to communicate meaning. For instance, red can signify urgency or error, while green often represents success or confirmation. Using the right color palette also ensures visual consistency across the interface. Check this amazing post of common color palettes, and also check out this color tool from Canva,com
- Typography: Fonts and typefaces play a crucial role in readability and user perception as it could be used to convey tone, from playful to professional, and establish visual hierarchy through font size, weight, and spacing.
- Interaction Design: This includes how buttons, menus, and other controls respond to clicks, taps, swipes, or other user inputs. It aims to make the interface intuitive and engaging.
- Brand Identity: All visual elements should align with the brand’s vision and style, creating a cohesive look that reflects the brand’s personality. Consistency in the use of colors, typography, imagery, and logos helps strengthen brand recognition. For example, an app for a bakery cannot use background images depicting the beaches, instead it may be better to use certain kind of color and images, and be consistent in its usage.
- Responsiveness: Ensures that the interface works well on various screen sizes and devices. This will help to provide an optimal user experience everywhere.
- Informational Components: Elements that provide users with information or feedback such as tooltips, notifications, progress bars, and pop-up messages
- Feedback Mechanisms: Indicate the result of user actions. It could be visual (animations, color changes), auditory (sounds), or tactile (vibrations). If a user interaction produces no observable result, the user could become confused even though the action was successful. For example, if you click a submit button, you would expect to see some slight color changes or any other visual result
Tkinter variables and Input Validation
Tkinter GUI has the ability to get inputs from the user, and these variables could exist as a string, boolean, integer or a floating numbers. The methods below helps to properly assign these variables
- StringVar(): These method helps to initialize the variable as a string data type
- IntVar(): These method helps to initialize the variable as an integer number data type
- DoubleVar(): These method helps to initialize the variable as a floating number type
- BooleanVar(): These method helps to initialize the variable as a boolean data type, usually used for widgets like check buttons or radio buttons which state is either selected or deselected
The above variables all have an optional attribute called value, that can be used to set default values. In addition to these tkinter variables we should also know how to automatically change the content present in the widget or get the values that was inputted. This brings us to some important methods that allow for dynamic content manipulation.
get
Method: Theget
method is used to retrieve the current value stored in a Tkinter variable, such asStringVar()
,IntVar()
,DoubleVar()
, orBooleanVar()
.
name_var = tb.StringVar(value="my name")
entered_name = name_var.get() # Retrieves the value stored in 'name_var'
set
Method: Theset
method is used to update or change the value of a Tkinter variable. It modifies the variable and automatically updates any associated widgets.
age_var = tb.IntVar()
age_var.set(25) # Sets the value of 'age_var' to 25
insert
Method: Theinsert
method is typically used withText
orScrolledText
widgets to insert text at a specified location.
text_widget.insert("1.0", "Hello, World!") # Inserts text at the beginning
delete
Method: Thedelete
method is used to remove a range of text from aText
orScrolledText
widget.
text_widget.delete("1.0", "end") # Deletes all text from the widget
Basic Tkinter Widgets
To get you fully started, we will do a quick review on the most commonly used widgets: Window, Frames, Label, Button, Entry, Scrolltext
Window: The window is the main container or root of a tkinter application, and it’s created by calling the Window()
function, which creates the main window where all other widgets can reside.
import tkinter as tk
root = tb.Window() # Creates the main window
root.title("Basic Tkinter Window")
root.mainloop() # Keeps the window open and responsive
Frame: A frame is used to group and organize other widgets, and are also useful for dividing the window into sections, allowing for more structured layout management. It’s a good idea to always create a main frame in which you would place the sub frames of other widgets
main_frame = tb.Frame(root, width=300, height=200)
main_frame.pack(fill='both', expand=True)
Label: The label widget is used to display text or images. It is a static widget, meaning it is used for displaying information rather than interacting with the user.
label = tb.Label(main_frame, text="Welcome to Tkinter!", font=("Arial", 16))
label.pack()
Button: A button widget allows users to trigger an action when clicked. You can associate a function to the button using the command
option.
button = tb.Button(main_frame, text="Click Me", command=button_click)
button.pack()
def button_click(): -> None
""" Function runs when button is clicked"
print("Button clicked!")
Entry: The entry widget is a single-line text field that allows the user to input data. It is commonly used for forms or collecting short input from users.
entry = tb.Entry(main_frame)
entry.pack(pady=5)entry.pack()
Text: The scrolled text widget is a text widget with an integrated scrollbar. It is useful for multi-line text inputs or displaying long text content.
text = tb.Text(main_frame, width=30, height=5)
text.pack()scroll_text.pack()
Exercise
- Two lines of code have been extracted from a file called main_v2.py in the project link above
- Explain the purpose of the adding a conditional block
- Give me more validation you think could be added to a field that gets users name
user_name = user_name_entry.get()
if len(user_name) > 0:
- Develop a GUI that helps the users to calculate and display the area and perimeter of a rectangle by just entering two real numbers and clicking submit
- Create a new file in the sandbox called BASICS (created last class).
- Let the new file be called “rectangle
- Set up the main window
- How many labels, entry field and buttons do you need.
- Based on your answers to the questions above create your GUI
Conclusion
In this lesson we explored the principles of GUI programming, with tkinter and ttkbootstrap software tools. We discussed components such as windows, frames, labels, buttons, input fields and text boxes. These elements form the foundation of any graphical user interface application. They enable us to develop programs that facilitate data viewing and input by users. Additionally you acquired knowledge about writing Python code in a step, by step manner and understanding ways to organize and control graphical user interface designs efficiently.