3 Object Oriented Programming (I)
Overview
Object Oriented Programming (OOP) is a programming style in python that focuses creating objects that contains both data (attributes) and methods that can operate on the data.
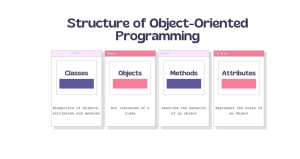
TEKS Computer Science 1 (3): To foster students’ creativity and innovation by presenting opportunities to design, implement, and present meaningful programs through a variety of media
Content Objective
- Students will create a simple graphical user interface using basic Tkinter widgets, including windows, frames, labels, buttons, entry fields, and textbox areas.
- Students will learn how Tkinter variables work and methods for input validation.
- Keywords (Phrases): Graphical User Interface, Widgets
Language Objective (read, write, speak):
- Students will be able to read and interpret Python code for GUI programming.
- Students will be able to write descriptive code comments explaining the purpose any logic used in their project
- Students will verbally explain the purpose of GUI components and their implementation in Python to their peers.
Key terms to take note of (Click here for link to code)
- Classes: A blueprint / template for creating objects. which have properties (attributes) and behaviors (methods/functions)
- Attributes: Attributes also known as properties are variables that belongs to a class and defines its characteristics for example age, name etc., There are two types of attributes.
- Class attributes– These variables are defined at the class level and are shared amongst all instances of the class, thus can be accessed from any instance or from the class itself.
- Instance attributes– These variables are defined within the constructor (__init__) method and its values are unique to each instance of a class
- Methods: Also known as behaviors are the functions within a class that defines some action or operations that an object can perform on the instance variable, and they help us to encapsulate the behavior or functionality of an object. For example, in tkinter these behaviors may be commands to make a button or widget to perform some action when clicked. In python we have 3 kinds of Methods
- Instance Methods– These functions operate on the instances of a class and its capable of accessing instance variables. It takes self as its first argument.
- Class Methods– These functions operate on the class itself including the class variables. It takes cls as its first argument
- Static Methods– These functions operate independently of the instance of class itself, and used as an utility functions or helper methods. It has no specific requirement for “self” or “cls” as first argument
- Objects: This refers to an instance of a class, with each instance containing the data and behavior defined by the class, as well as a unique identity.
- Inheritance: Inheritance allows a programmer to create a new class based on an existing class, thus inheriting the instance attributes and instance methods of the parent class
- Encapsulation: Encapsulation is the process of dividing your program into smaller units with each unit internally operating independently of the others. The pieces interact with each other, but they don’t need to know exactly how each one accomplishes its tasks. In the code for example, we could have two different Bus instances, each having a unique name or speed that can be managed with ease
- Data Abstraction: Data abstraction involves separating the interface (what an object can do) and implementation(how the object does this internally). For example, we all know
that Math.abs() returns the absolute value of a number, but we do not know how it was implemented
Guided Exercise
- Create 2 instance object each from the Motorcycle, Car, and Truck Class
- Add at least on extra method You could add to the Car and Truck Class
- Create a new class called Airplane that inherits from the Vehicle class
Exercise
- Write a class called Investment with fields called principal and interest. The constructor should set the values of those fields. There should be a method called value_after that
returns the value of the investment after n years. The formula for this is p(1 + i)n, where p is the principal, and i is the interest rate. It should also use the special method __str__ so that
printing the object will result in something like below:
Principal – $1000.00, Interest rate – 5.12% - Write a class
ElectricityBill
with fieldsunits_consumed
andrate_per_unit
.- Add a method
calculate_bill()
that calculates the bill using the formula:
- Override the
__str__
method to display:
“Units Consumed: 450, Rate Per Unit: $0.12, Total Bill: $54.00”
- Add a method
- Write a class
Rectangle
with fieldslength
andwidth
.- Add methods:
area()
to calculate and return the area of the rectangle.perimeter()
to calculate and return the perimeter of the rectangle.
- Override the
__str__
method to display:
“Rectangle: Length = 10, Width = 5, Area = 50, Perimeter = 30”
- Add methods:
- Write a class
BankAccount
with fieldsaccount_holder
,balance
, andinterest_rate
.
Add the following methods:deposit(amount)
to increase the balance.withdraw(amount)
to decrease the balance (ensure balance does not go negative).apply_interest()
to calculate and add interest based on theinterest_rate
.
- Override the
__str__
method to display:
“Account Holder: John Doe, Balance: $2000.00, Interest Rate: 1.5%”
Ensure that balance never becomes negative.
- Pick a real-world object and design a class for it (e.g., a
Library
orSmartphone
orAnimal
class).
Conclusion
We have concluded the basics of object oriented programming in python, we have learned about methods, properties, variables , encapsulation, inheritance, and their relevance in making your more structured